含电路图和源程序
http://www.dianyuan.com/blog/blog_comment_list.php?blar_id=77970
我的电源网博客新开张,希望大家有空去逛逛!!
谢谢!
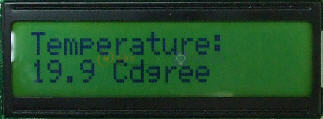
500) {this.resized=true; this.width=500; this.alt='这是一张缩略图,点击可放大。\n按住CTRL,滚动鼠标滚轮可自由缩放';this.style.cursor='hand'}" onclick="if(!this.resized) {return true;} else {window.open('http://u.dianyuan.com/bbs/u/61/163911199240652.jpg?x-oss-process=image/watermark,g_center,image_YXJ0aWNsZS9wdWJsaWMvd2F0ZXJtYXJrLnBuZz94LW9zcy1wcm9jZXNzPWltYWdlL3Jlc2l6ZSxQXzQwCg,t_20');}" onmousewheel="return imgzoom(this);">